All Packages Class Hierarchy This Package Previous Next Index
Class jclass.table.JCTable
java.lang.Object
|
+----java.awt.Component
|
+----java.awt.Container
|
+----java.awt.Panel
|
+----jclass.table.Table
|
+----jclass.table.JCTable
- public class JCTable
- extends Table
JCTable enables the following functionality:
- greater general flexibility in design, and the look-and-feel of a table
- makes it easier for users to find information with built-in search
and sorting features
- mix fonts in your tables to enhance and emphasize text
- change the color of fonts and backgrounds
- control margin spacing within cells, and the spaces between cells
- change borders styles for your table
- change cell styles, so that cells, rows or columns appear raised, inset
or framed
- add shadow effects
- a cell, row or column can be dragged onscreen to create to span other
cells, rows or columns
- allow users to search for specific information in your table
- display large tables in a scrollable form, enabling users to conveniently
view information without having to resize their display
- add pictures, text and hypertext links within a single cell
- change the types and styles of cell and table borders.

JCTable()
- Creates a table.
JCTable(Applet,
String)
- Creates a table which reads parameters from an HTML file.

addColumn(int,
Object, Vector)
- Inserts a new column into the table, shifting any cell values and attributes
(such as background color) to the right of the insertion.
addRow(int,
Object, Vector)
- Inserts a new row into the table, shifting any cell values and attributes
(such as background color) below the insertion.
cancelEdit(boolean)
- Cancels interactive editing of a cell and, as an option, hides the
Text component.
clearCells()
- Sets clears the values of all cells by setting them to null.
clearSelectedCells()
- All selected cells are unselected.
commitEdit(boolean)
- Commits interactive cell editing internally, saving any changes made
by the user.
deleteColumns(int,
int)
- Deletes columns from the table.
deleteRows(int,
int)
- Deletes rows from the table.
eventToCell(Event)
- Determines the cell or label in which an event occurred.
findSpan(int,
int, JCCellRange, Dimension)
- Finds the first range which the specified cell or label spans.
getAllowCellResize()
- Gets the table's AllowCellResize value.
getBounds(int,
int, Rectangle)
- Gets the bounding rectangle of a cell or label.
getCallback(int)
- Gets the callback object for the specified reason.
getCell(int,
int)
- This gets the value of a cell or label.
getCells()
- Returns the internal cell values.
getColumnLabel(int)
- This gets a column label.
getColumnLabelOffset()
- Gets the table's ColumnLabelOffset value.
getColumnLabelPlacement()
- Gets the table's ColumnLabelPlacement value.
getColumnLabels()
- Gets the table's ColumnLabels value.
getColumnLabelSort()
- Gets the table's ColumnLabelSort value.
getComponent(int,
int)
- Locates the component currently displayed in the cell, and returns
null if there is no component in the cell or if the component is not currently
displayed.
getCurrentCell()
- Determines the cell being edited.
getDisplayClipArrows()
- Gets the table's DisplayClipArrows value.
getDoubleBuffer()
- Gets the table's DoubleBuffer value.
getEditable(int,
int)
- Returns the editable value for a cell.
getFrameBorderType()
- Gets the table's FrameBorderType value.
getFrameShadowThickness()
- Gets the table's FrameShadowThickness value.
getFrozenColumnPlacement()
- Gets the table's FrozenColumnPlacement value.
getFrozenColumns()
- Gets the table's FrozenColumns value.
getFrozenRowPlacement()
- Gets the table's FrozenRowPlacement value.
getFrozenRows()
- Gets the table's FrozenRows value.
getHorizSBAttachment()
- Gets the table's HorizSBAttachment value.
getHorizSBDisplay()
- Gets the table's HorizSBDisplay value.
getHorizSBOffset()
- Gets the table's HorizSBOffset value.
getHorizSBPosition()
- Gets the table's HorizSBPosition value.
getJumpScroll()
- Gets the table's JumpScroll value.
getLeftColumn()
- Gets the table's LeftColumn value.
getMarginHeight()
- Gets the table's MarginHeight value.
getMarginWidth()
- Gets the table's MarginWidth value.
getMaxLength(int,
int)
- Returns the maxLength value for a cell.
getMinCellVisibility()
- Gets the table's MinCellVisibility value.
getMode()
- Gets the table's Mode value.
getMultiline(int,
int)
- Returns the multiline value for a cell.
getNumColumns()
- Gets the table's NumColumns value.
getNumRows()
- Gets the table's NumRows value.
getParameters(Applet)
- Reads the parameter values from the HTML page using the specified applet.
getParameters(Applet,
String)
- Reads the parameter values from the file.
getPosition(Component,
JCCellPosition)
- Finds the location of any component set in a cell/label.
getRepaint()
- Gets the table's Repaint value.
getRepeatBackgroundColors()
- Gets the table's RepeatBackgroundColors value.
getRepeatForegroundColors()
- Gets the table's RepeatForegroundColors value.
getRowLabel(int)
- This gets a row label.
getRowLabelOffset()
- Gets the table's RowLabelOffset value.
getRowLabelPlacement()
- Gets the table's RowLabelPlacement value.
getRowLabels()
- Gets the table's RowLabels value.
getSelectedBackground()
- Gets the table's SelectedBackground value.
getSelectedCells()
- Gets the table's SelectedCells value.
getSelectedForeground()
- Gets the table's SelectedForeground value.
getSelectedRange(int,
JCCellRange)
- Retrieves a currently-selected range from the SelectedCellList.
getSelectionPolicy()
- Gets the table's SelectionPolicy value.
getShadowThickness()
- Gets the table's ShadowThickness value.
getSpans()
- Gets the table's Spans value.
getStringCase(int,
int)
- Returns the stringCase value for a cell.
getTextComponent()
- Returns the currently displayed Text component.
getTopRow()
- Gets the table's TopRow value.
getTrackCursor()
- Gets the table's TrackCursor value.
getTraversable(int,
int)
- Returns the traversable value for a cell.
getUserdata(int,
int)
- Returns the userdata object for a cell or label.
getValidatePolicy()
- Gets the table's ValidatePolicy value.
getVertSBAttachment()
- Gets the table's VertSBAttachment value.
getVertSBDisplay()
- Gets the table's VertSBDisplay value.
getVertSBOffset()
- Gets the table's VertSBOffset value.
getVertSBPosition()
- Gets the table's VertSBPosition value.
getVisibleColumns()
- Gets the table's VisibleColumns value.
getVisibleRows()
- Gets the table's VisibleRows value.
hasCallback(int)
- Returns true if a callback has been registered for the specified reason.
isCell(int,
int)
- If key is LEFT, RIGHT, DOWN, UP, TAB, or SHIFT-TAB, the adjacent cell
is traversed to.
isColumnLabel(int,
int)
- This returns true if the row and column constitute a valid column label.
isLabel(int,
int)
- This returns true if the row and column constitute a valid label.
isRowLabel(int,
int)
- This returns true if the row and column constitute a valid row label.
isSelected(int,
int)
- Determines whether the cell is selected.
isTraversable(int,
int)
- Returns true if the cell can be traversed to -- in other words, if
its traverse property is true, and is not spanned by another cell.
isVisible(int,
int)
- This checks whether a cell is visible.
keyDown(Event,
int)
- If key is PGDN or PGUP, the table is scrolled.
makeColumnVisible(int)
- This scrolls a column so it is visible on the screen.
makeRowVisible(int)
- This scrolls a row so it is visible on the screen.
makeVisible(int,
int)
- This scrolls a frozen or non-frozen cell or label so it is visible
on the screen.
mouseExit(Event,
int, int)
- This tracks the cursor if TrackCursor is true.
mouseDown(Event,
int, int)
- If the SHIFT key or CONTROL key is pressed, a new range is selected.
mouseDrag(Event,
int, int)
- Resizes a cell or selects it.
mouseMove(Event,
int, int)
- This tracks the cursor if TrackCursor is true.
mouseUp(Event,
int, int)
- Resizes a cell or selects it.
moveColumns(int,
int, int)
- Moves a range of columns.
moveRows(int,
int, int)
- Moves a range of rows.
preferredSize()
- Returns the preferred size of the component.
setAlignment(int,
int, int)
- Sets the alignment of cell/label text and/or image.
setAllowCellResize(int)
- Sets how an end-user can interactively resize rows/columns.
setBackground(int,
int, Color)
- Sets the background color of cells and labels.
setBackground(int,
int, int)
- Allows the background color of a cell or label to be set to JCTblEnum.REPEAT_ROW
or JCTblEnum.REPEAT_COLUMN.
setBorderSides(int,
int, int)
- Sets the sides of a cell or label that display the border type in their
border area.
setBorderType(int,
int, int)
- Sets the style used for cell and label borders.
setCallback(int,
JCTblCallback)
- Sets the callback object for the specified reason.
setCell(int,
int, Object)
- Sets the value of a cell or label.
setCells(String[][])
- Sets the cell values as a matrix of Strings. To remove all values,
call clearCells().
setCells(Vector)
- Sets the cell values as a Vector of Vectors.
setCharHeight(int,
int)
- Sets the number of lines of text that a row can display (default: 1).
setCharWidth(int,
int)
- Sets the number of characters that a column can display (default: 10).
setColumnLabel(int,
Object)
- Sets a column label.
setColumnLabelOffset(int)
- Sets the spacing between the column labels and the cell area in pixels
(default: 0).
setColumnLabelPlacement(int)
- Sets the position of the column labels relative to the cells.
setColumnLabels(String[])
- Sets the column labels as an array of Strings.
setColumnLabels(Vector)
- Sets the column labels as a Vector.
setColumnLabelSort(boolean)
- Specifies whether or not a column is sorted when its label is clicked
(default: false).
setComponent(int,
int, Component)
- Sets the component for a cell or label.
setDatatype(int,
int, int)
- Sets the data type of a cell/label value.
setDisplayClipArrows(boolean)
- Sets whether or not to display clipping arrows when a cell/label value
cannot be fully displayed in its cell/label (default: true).
setDoubleBuffer(boolean)
- Controls whether or not double-buffering is used when displaying and
updating the table.
setEditable(int,
int, boolean)
- Sets whether cell values can be changed by an end-user (default: true).
setFont(int,
int, Font)
- Sets the font to be used to display cell and/or label values.
setForeground(int,
int, Color)
- Sets the foreground color (the text color) of cells and labels.
setForeground(int,
int, int)
- Allows the foreground color of a cell or label to be set to JCTblEnum.REPEAT_ROW
or JCTblEnum.REPEAT_COLUMN.
setFrameBorderType(int)
- Sets the type of border for the frame surrounding the cell and label
areas (default: JCTblEnum.BORDER_IN).
setFrameShadowThickness(int)
- Sets the thickness of the frame shadows surrounding the cell and label
areas (default: 0).
setFrozenColumnPlacement(int)
- Sets the location of all frozen columns within the component display.
setFrozenColumns(int)
- Sets the number of columns from the start of the table that are not
horizontally scrollable (default: 0).
setFrozenRowPlacement(int)
- Sets the location of all frozen rows.
setFrozenRows(int)
- Sets the number of rows from the start of the table that are not vertically
scrollable (default: 0).
setHorizSBAttachment(int)
- Sets how the end of the horizontal scrollbar is attached to the table.
setHorizSBDisplay(int)
- Sets when to display the horizontal scrollbar.
setHorizSBOffset(int)
- Sets the distance between the horizontal scrollbar and the table (default:
6).
setHorizSBPosition(int)
- Sets how the horizontal scrollbar is attached to the table.
setJumpScroll(int)
- Sets the scrolling behavior of each scrollbar.
setLeftColumn(int)
- Sets the non-frozen column at least partially visible at the left side
of the window.
setMarginHeight(int)
- Sets the distance (in pixels) between the inside edge of the cell border
and the top and bottom edge of the cell's contents (default: 2).
setMarginWidth(int)
- Sets the distance (in pixels) between the inside edge of the cell border
and the left/right edge of the cell's contents (default: 5).
setMaxLength(int,
int, int)
- Sets the maximum number of characters that can be entered in a cell
by a user (default: MAXINT).
setMinCellVisibility(int)
- Sets the minimum amount of a cell that is made visible when edited.
setMode(int)
- Sets the display mode.
JCTblEnum.MODE_LIST list operation
JCTblEnum.MODE_TABLE table operates normally
When this property is set to MODE_LIST, selecting a cell causes all
cells in the row to be selected.
setMultiline(int,
int, boolean)
- Sets whether or not a user can enter multiple lines of text into a
cell (that is, whether the Text component's editMode property is set to
MULTI_LINE_EDIT) (default: false).
setNumColumns(int)
- Sets the current number of columns in the table (default: 5).
setNumRows(int)
- Sets the current number of rows in the table (default: 10).
setPixelHeight(int,
int)
- Sets a row height in pixels.
setPixelWidth(int,
int)
- Sets a column width in pixels.
setRepaint(boolean)
- Sets whether the table should be redrawn and recomputed whenever one
of its properties is set (default: true).
setRepeatBackgroundColors(Color[])
- An array of colors to be used when a Background value is JCTblEnum.REPEAT_ROW
or JCTblEnum.REPEAT_COLUMN.
setRepeatForegroundColors(Color[])
- An array of colors to be used when a Foreground value is JCTblEnum.REPEAT_ROW
or JCTblEnum.REPEAT_COLUMN.
setRowLabel(int,
Object)
- Sets a row label.
setRowLabelOffset(int)
- Sets the spacing between the row labels and the cell area in pixels
(default: 0).
setRowLabelPlacement(int)
- Sets the position of the row labels relative to the cells.
setRowLabels(String[])
- Sets the row labels as an array of Strings.
setRowLabels(Vector)
- Sets the row labels as a Vector.
setSelectedBackground(Color)
- Sets the background color of selected cells (default: cell's foreground
color).
setSelectedCells(JCCellRange)
- Sets a range of selected cells.
setSelectedCells(Vector)
- Sets an array of currently-selected ranges of cells.
setSelectedForeground(Color)
- Sets the foreground color of selected cells (default: cell's background
color).
setSelectionPolicy(int)
- Sets the selection behavior allowed on the table, both by end-users,
and by the application.
setShadowThickness(int)
- Sets the thickness of the shadows surrounding each cell and label (default:
1).
setSpans(Vector)
- Sets an array of currently-spanned ranges of cells or labels.
setStringCase(int,
int, int)
- Sets the case of cell values of cells edited by the user.
setTopRow(int)
- Sets the non-frozen row at least partially visible at the top of the
window.
setTrackCursor(boolean)
- Sets whether the mouse pointer should be tracked as a user moves the
mouse across the table.
setTraversable(int,
int, boolean)
- Sets whether the cell can be traversed to (default: true).
setUserdata(int,
int, Object)
- Sets a user data object that the application can attach.
setValidatePolicy(int)
- Sets when to validate changes to cell or label values against its data
type (specified by DataType).
setVertSBAttachment(int)
- Sets the attachment of the vertical scrollbar to the table.
setVertSBDisplay(int)
- Sets when to display the vertical scrollbar.
setVertSBOffset(int)
- Sets the distance between the vertical scrollbar and the table (default:
6).
setVertSBPosition(int)
- Sets attachment of the horizontal scrollbar to the table.
setVisibleColumns(int)
- Sets the number of columns currently visible or partially visible.
setVisibleRows(int)
- Sets the number of rows currently visible or partially visible.
sortByColumn(int,
JCSortInterface)
- Sorts the rows in the table based on a specified column, using the
String equivalents of cell values.
traverse(int,
int, boolean, boolean)
- Attempts to move the current cell focus to a new location.

JCTable
public JCTable()
- Creates a table. No parameters are read from a HTML file.
JCTable
public JCTable(Applet applet,
String name)
- Creates a table which reads parameters from the applet's HTML file.
- Parameters:
- applet - applet whose PARAM tags are to be read
- name - if not null, only the parameters preceded by this name are read
- See Also:
- getParameter
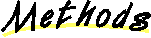
getCallback
public JCTblCallback getCallback(int reason)
- Gets the callback object for the specified reason.
- Returns:
- null if no callback has been registered
- See Also:
- JCTblCallback,
setCallback
hasCallback
public boolean hasCallback(int reason)
- Returns true if a callback has been registered for the specified reason.
- Returns:
- false if no callback has been registered
- See Also:
- JCTblCallback,
setCallback
isCell
public static boolean isCell(int row,
int col)
This returns true if the row and column constitute a valid cell.
isLabel
public static boolean isLabel(int row,
int col)
- This returns true if the row and column constitute a valid label.
isColumnLabel
public static boolean isColumnLabel(int row,
int col)
- This returns true if the row and column constitute a valid column label.
isRowLabel
public static boolean isRowLabel(int row,
int col)
- This returns true if the row and column constitute a valid row label.
setCallback
public void setCallback(int reason,
JCTblCallback cb)
- Sets the callback object for the specified reason. The callback object
must implement the JCTblCallback interface.
- Parameters:
- reason - one of the JCTblCallback reasons
- cb - the callback instance; if null, any previously registered callback
is removed.
- See Also:
- JCTblCallback
getAllowCellResize
public int getAllowCellResize()
- Gets the table's AllowCellResize value.
- See Also:
- setAllowCellResize
setAllowCellResize
public void setAllowCellResize(int v)
- Sets how an end-user can interactively resize rows/columns. Valid values
are:
- JCTblEnum.RESIZE_ALL (default)
- JCTblEnum.RESIZE_NONE
- JCTblEnum.RESIZE_COLUMN
- JCTblEnum.RESIZE_ROW
getDisplayClipArrows
public boolean getDisplayClipArrows()
- Gets the table's DisplayClipArrows value.
- See Also:
- setDisplayClipArrows
setDisplayClipArrows
public void setDisplayClipArrows(boolean v)
- Sets whether or not to display clipping arrows when a cell/label value
cannot be fully displayed in its cell/label (default: true). Clipping arrows
appear at the bottom-right corner of a cell/label.
getDoubleBuffer
public boolean getDoubleBuffer()
- Gets the table's DoubleBuffer value.
- See Also:
- setDoubleBuffer
setDoubleBuffer
public void setDoubleBuffer(boolean v)
- Controls whether or not double-buffering is used when displaying and
updating the table.
getFrameBorderType
public int getFrameBorderType()
- Gets the table's FrameBorderType value.
- See Also:
- setFrameBorderType
setFrameBorderType
public void setFrameBorderType(int v)
- Sets the type of border for the frame surrounding the cell and label
areas (default: JCTblEnum.BORDER_IN). Possible values are:
- JCTblEnum.BORDER_NONE
- JCTblEnum.BORDER_ETCHED_IN
- JCTblEnum.BORDER_ETCHED_OUT
- JCTblEnum.BORDER_IN
- JCTblEnum.BORDER_OUT
- JCTblEnum.BORDER_PLAIN
FrameShadowThickness must be set for the border to be visible.
- See Also:
- setFrameShadowThickness
getFrameShadowThickness
public int getFrameShadowThickness()
- Gets the table's FrameShadowThickness value.
- See Also:
- setFrameShadowThickness
setFrameShadowThickness
public void setFrameShadowThickness(int v)
- Sets the thickness of the frame shadows surrounding the cell and label
areas (default: 0).
- See Also:
- setFrameBorderType
getFrozenColumnPlacement
public int getFrozenColumnPlacement()
- Gets the table's FrozenColumnPlacement value.
- See Also:
- setFrozenColumnPlacement
setFrozenColumnPlacement
public void setFrozenColumnPlacement(int v)
- Sets the location of all frozen columns within the component display.
Changing the placement of frozen columns does not change the location of
the columns in the table's internal CellValues: JCTblEnum.PLACE_LEFT or
JCTblEnum.PLACE_RIGHT.
getFrozenColumns
public int getFrozenColumns()
- Gets the table's FrozenColumns value.
- See Also:
- setFrozenColumns
setFrozenColumns
public void setFrozenColumns(int v)
- Sets the number of columns from the start of the table that are not
horizontally scrollable (default: 0). Placement of the frozen columns on
the screen is specified by FrozenColumnPlacement.
getFrozenRowPlacement
public int getFrozenRowPlacement()
- Gets the table's FrozenRowPlacement value.
- See Also:
- setFrozenRowPlacement
setFrozenRowPlacement
public void setFrozenRowPlacement(int v)
- Sets the location of all frozen rows. Changing the placement of frozen
rows does not change the location of the rows in the table's internal CellValues.
Valid values are JCTblEnum.PLACE_TOP and JCTblEnum.PLACE_BOTTOM.
getFrozenRows
public int getFrozenRows()
- Gets the table's FrozenRows value.
- See Also:
- setFrozenRows
setFrozenRows
public void setFrozenRows(int v)
- Sets the number of rows from the start of the table that are not vertically
scrollable (default: 0). Placement of the frozen rows on the screen is
specified by FrozenRowPlacement.
getJumpScroll
public int getJumpScroll()
- Gets the table's JumpScroll value.
- See Also:
- setJumpScroll
setJumpScroll
public void setJumpScroll(int v)
- Sets the scrolling behavior of each scrollbar. Scrollbars can either
scroll smoothly or "jump" scroll in whole row/column increments.
When set to JCTblEnum.JUMP_NONE, both scrollbars will scroll smoothly.
When set to JCTblEnum.JUMP_HORIZONTAL, the horizontal scrollbar jump scrolls.
When set to JCTblEnum.JUMP_VERTICAL, the vertical scrollbar jump scrolls.
When set to JCTblEnum.JUMP_ALL, both scrollbars jump scroll.
getLeftColumn
public int getLeftColumn()
- Gets the table's LeftColumn value.
- See Also:
- setLeftColumn
setLeftColumn
public void setLeftColumn(int v)
- Sets the non-frozen column at least partially visible at the left side
of the window. This value is updated as a user scrolls through a table.
When set, the table scrolls to display the column at the left side of the
window. This value is always larger or equal to the number of frozen columns.
getMarginHeight
public int getMarginHeight()
- Gets the table's MarginHeight value.
- See Also:
- setMarginHeight
setMarginHeight
public void setMarginHeight(int v)
- Sets the distance (in pixels) between the inside edge of the cell border
and the top and bottom edge of the cell's contents (default: 2).
getMarginWidth
public int getMarginWidth()
- Gets the table's MarginWidth value.
- See Also:
- setMarginWidth
setMarginWidth
public void setMarginWidth(int v)
- Sets the distance (in pixels) between the inside edge of the cell border
and the left/right edge of the cell's contents (default: 5). This property
affects the entire table.
getHorizSBAttachment
public int getHorizSBAttachment()
- Gets the table's HorizSBAttachment value.
- See Also:
- setHorizSBAttachment
setHorizSBAttachment
public void setHorizSBAttachment(int v)
- Sets how the end of the horizontal scrollbar is attached to the table.
When set to JCTblEnum.ATTACH_CELLS (default), the scrollbar ends at the
edge of the visible cells. When set to JCTblEnum.ATTACH_SIDE, the scrollbar
ends at the edge of the table.
getHorizSBPosition
public int getHorizSBPosition()
- Gets the table's HorizSBPosition value.
- See Also:
- setHorizSBPosition
setHorizSBPosition
public void setHorizSBPosition(int v)
- Sets how the horizontal scrollbar is attached to the table. When set
to JCTblEnum.SBPOSITION_CELLS (default), the scrollbar is attached to the
cell/label viewport. When set to JCTblEnum.SBPOSITION_SIDE, the scrollbar
is attached to the side of the table.
getHorizSBOffset
public int getHorizSBOffset()
- Gets the table's HorizSBOffset value.
- See Also:
- setHorizSBOffset
setHorizSBOffset
public void setHorizSBOffset(int v)
- Sets the distance between the horizontal scrollbar and the table (default:
6).
getHorizSBDisplay
public int getHorizSBDisplay()
- Gets the table's HorizSBDisplay value.
- See Also:
- setHorizSBDisplay
setHorizSBDisplay
public void setHorizSBDisplay(int v)
- Sets when to display the horizontal scrollbar. Valid values are:
JCTblEnum.SBDISPLAY_ALWAYS always displayed
JCTblEnum.SBDISPLAY_NEVER never displayed
JCTblEnum.SBDISPLAY_AS_NEEDED displayed when the rows or columns are not visible
getVertSBAttachment
public int getVertSBAttachment()
- Gets the table's VertSBAttachment value.
- See Also:
- setVertSBAttachment
setVertSBAttachment
public void setVertSBAttachment(int v)
- Sets the attachment of the vertical scrollbar to the table. When set
to JCTblEnum.ATTACH_CELLS (default), the scrollbar ends at the edge of
the visible cells. When set to JCTblEnum.ATTACH_SIDE, the scrollbar ends
at the edge of the table.
getVertSBPosition
public int getVertSBPosition()
- Gets the table's VertSBPosition value.
- See Also:
- setVertSBPosition
setVertSBPosition
public void setVertSBPosition(int v)
- Sets attachment of the horizontal scrollbar to the table. When set
to JCTblEnum.SBPOSITION_CELLS (default), the scrollbar is attached to the
cell/label viewport. When set to JCTblEnum.SBPOSITION_SIDE, the scrollbar
is attached to the side of the table.
getVertSBOffset
public int getVertSBOffset()
- Gets the table's VertSBOffset value.
- See Also:
- setVertSBOffset
setVertSBOffset
public void setVertSBOffset(int v)
- Sets the distance between the vertical scrollbar and the table (default:
6).
getVertSBDisplay
public int getVertSBDisplay()
- Gets the table's VertSBDisplay value.
- See Also:
- setVertSBDisplay
setVertSBDisplay
public void setVertSBDisplay(int v)
- Sets when to display the vertical scrollbar. Valid values are:
JCTblEnum.SBDISPLAY_ALWAYS always displayed
JCTblEnum.SBDISPLAY_NEVER never displayed
JCTblEnum.SBDISPLAY_AS_NEEDED displayed when the rows or columns are not visible
getMinCellVisibility
public int getMinCellVisibility()
- Gets the table's MinCellVisibility value.
- See Also:
- setMinCellVisibility
setMinCellVisibility
public void setMinCellVisibility(int v)
- Sets the minimum amount of a cell that is made visible when edited.
When the table scrolls to edit a non-visible cell, this property determines
the percentage of the cell scrolled into view. When set to 100, the cell
is made completely visible. When set to 10, only 10% of the cell is made
visible. If set to 0, the cell will not scroll to be made visible. This
property affects the behavior of makeVisible().
getMode
public int getMode()
- Gets the table's Mode value.
- See Also:
- setMode
setMode
public void setMode(int v)
- Sets the display mode.
JCTblEnum.MODE_LIST list operation
JCTblEnum.MODE_TABLE table operates normally
When this property is set to MODE_LIST, selecting a cell causes all
cells in the row to be selected. In addition, the following resources are
set:
- HorizSBAttachment and VertSBAttachment are set to ATTACH_SIDE
- HorizSBPosition and VertSBPosition are set to SBPOSITION_SIDE
- SelectionPolicy is set to SELECT_SINGLE
- ShadowThickness is set to 0
- Cell traversal is disallowed
The SelectionPolicy value controls whether users are allowed to select
single rows, a range of rows, or multiple ranges of rows.
- See Also:
- setHorizSBAttachment, setHorizSBPosition,
setSelectionPolicy, setShadowThickness,
setTraversable, setVertSBAttachment,
setVertSBPosition
getNumColumns
public int getNumColumns()
- Gets the table's NumColumns value.
- See Also:
- setNumColumns
setNumColumns
public void setNumColumns(int v)
- Sets the current number of columns in the table (default: 5). This
value does not affect the table's internal CellValues vector. JCTable.addColumn()
and JCTable.deleteColumns() update this property by the number of columns
added/deleted. If set to zero, no cells are displayed.
getNumRows
public int getNumRows()
- Gets the table's NumRows value.
- See Also:
- setNumRows
setNumRows
public void setNumRows(int v)
- Sets the current number of rows in the table (default: 10). This value
does not affect the table's internal CellValues vector. JCTable.addRow()
and JCTable.deleteRow() update this property by the number of rows added/deleted.
If set to zero, no cells are displayed.
getParameters
public void getParameters(Applet applet)
- Reads the parameter values from the HTML page using the specified applet.
The values will override those previously set.
- See Also:
- getParameter
getParameters
public void getParameters(Applet applet,
String file)
- Reads the parameter values from the file. The values will override
those previously set.
- Parameters:
- applet - if not null and in a browser, its documentBase() is used to
construct a complete filename if necessary.
- file - if an http protocol is not specified (if a ":" is
not present), the current working directory is used.
getRepaint
public boolean getRepaint()
- Gets the table's Repaint value.
- See Also:
- setRepaint
setRepaint
public void setRepaint(boolean v)
- Sets whether the table should be redrawn and recomputed whenever one
of its properties is set (default: true). If false, setting a property
will have no effect until repaint is switched to true.
getRepeatBackgroundColors
public Color[] getRepeatBackgroundColors()
- Gets the table's RepeatBackgroundColors value.
- See Also:
- setRepeatBackgroundColors
setRepeatBackgroundColors
public void setRepeatBackgroundColors(Color v[])
- An array of colors to be used when a Background value is JCTblEnum.REPEAT_ROW
or JCTblEnum.REPEAT_COLUMN.
getRepeatForegroundColors
public Color[] getRepeatForegroundColors()
- Gets the table's RepeatForegroundColors value.
- See Also:
- setRepeatForegroundColors
setRepeatForegroundColors
public void setRepeatForegroundColors(Color v[])
- An array of colors to be used when a Foreground value is JCTblEnum.REPEAT_ROW
or JCTblEnum.REPEAT_COLUMN.
getSelectedBackground
public Color getSelectedBackground()
- Gets the table's SelectedBackground value.
- See Also:
- setSelectedBackground
setSelectedBackground
public void setSelectedBackground(Color v)
- Sets the background color of selected cells (default: cell's foreground
color). When set to null, selected cells look identical to unselected cells
- the background and foreground colors are the same as the colors defined
for the cells (Foreground and Background).
getSelectedCells
public Vector getSelectedCells()
- Gets the table's SelectedCells value.
- See Also:
- setSelectedCells
clearSelectedCells
public void clearSelectedCells()
- All selected cells are unselected.
- See Also:
- setSelectedCells
setSelectedCells
public void setSelectedCells(Vector v)
- Sets an array of currently-selected ranges of cells. Each element of
the vector is an instance of a JCCellRange. This value is updated dynamically
as a user selects cells. The selection policy controls the amount of selection
allowed on the table, both by users and by the application. Users can select
in any direction, so start_row and/or start_column may be greater than
end_row and/or end_column. When a user clicks a row/column label to select
an entire row or column, end_row or end_column are set to MAXINT.
Use JCTable.getSelectedRange() to conveniently retrieve one range from
the selected range list.
To unselect all cells, call clearSelectedCells.
- See Also:
- getSelectedRange, JCCellRange,
clearSelectedCells
setSelectedCells
public void setSelectedCells(JCCellRange r)
- Sets a range of selected cells. To unselect all cells, call clearSelectedCells.
- See Also:
- clearSelectedCells
getSelectedForeground
public Color getSelectedForeground()
- Gets the table's SelectedForeground value.
- See Also:
- setSelectedForeground
setSelectedForeground
public void setSelectedForeground(Color v)
- Sets the foreground color of selected cells (default: cell's background
color). If set to null when SelectedBackground is not null, the foreground
of selected cells is the same as the foreground colors defined for the
cells (Foreground). This value is ignored when SelectedBackground is set
to null.
getSelectedRange
public boolean getSelectedRange(int pos,
JCCellRange range)
- Retrieves a currently-selected range from the SelectedCellList.
- Parameters:
- pos - this is the position within the selected cell list
- range - this is the returned range, rationalized to read from top to
bottom and from left to right. Special values such as MAXINT are converted
to valid range values.
- Returns:
- false if no cells are selected, or any argument is invalid
getSelectionPolicy
public int getSelectionPolicy()
- Gets the table's SelectionPolicy value.
- See Also:
- setSelectionPolicy
setSelectionPolicy
public void setSelectionPolicy(int v)
- Sets the selection behavior allowed on the table, both by end-users,
and by the application. Valid values are:
JCTblEnum.SELECT_NONE No selection is allowed
JCTblEnum.SELECT_SINGLE Selection of only one cell allowed
JCTblEnum.SELECT_RANGE Selection of only one range of cells allowed
JCTblEnum.SELECT_MULTIRANGE Selection of multiple ranges of cells allowed
When SelectionPolicy is set to JCTblEnum.SELECT_NONE, SELECT callbacks
are not called as a user edits or attempts to select cells. Setting this
property does not change the selected cell list.
getShadowThickness
public int getShadowThickness()
- Gets the table's ShadowThickness value.
- See Also:
- setShadowThickness
setShadowThickness
public void setShadowThickness(int v)
- Sets the thickness of the shadows surrounding each cell and label (default:
1).
getSpans
public Vector getSpans()
- Gets the table's Spans value.
- See Also:
- setSpans
setSpans
public void setSpans(Vector v)
- Sets an array of currently-spanned ranges of cells or labels. Each
element of the vector is an instance of a JCCellRange. A spanned range
is a range of cells or labels that appear joined and can be treated as
one cell. The top-left cell (specified by the start_row and start_column
members) is the source cell for the spanned range. The cell/label value
and attributes of the source cell are displayed in the spanned cell. Attributes
for the spanned range must be set on the source cell. A spanned range cannot
include both cells and labels. There must also be more than one cell/label
in a spanned range; when a single-cell range is specified, it is removed
from the list.
- See Also:
- JCCellRange
getTopRow
public int getTopRow()
- Gets the table's TopRow value.
- See Also:
- setTopRow
setTopRow
public void setTopRow(int v)
- Sets the non-frozen row at least partially visible at the top of the
window. This value is updated as a user scrolls through a table. When set,
the table scrolls to display the row at the top of the window.
getTrackCursor
public boolean getTrackCursor()
- Gets the table's TrackCursor value.
- See Also:
- setTrackCursor
setTrackCursor
public void setTrackCursor(boolean v)
- Sets whether the mouse pointer should be tracked as a user moves the
mouse across the table. When true, JCTable tracks the mouse pointer and
changes its shape to reflect the interactions available to the user at
location. When set to false, the table uses the application-defined pointer.
getValidatePolicy
public int getValidatePolicy()
- Gets the table's ValidatePolicy value.
- See Also:
- setValidatePolicy
setValidatePolicy
public void setValidatePolicy(int v)
- Sets when to validate changes to cell or label values against its data
type (specified by DataType). When set to JCTblEnum.VALIDATE_USER_EDIT,
only changes made by a user are validated. When set to JCTblEnum.VALIDATE_SET,
only changes made by an application are validated. When set to JCTblEnum.VALIDATE_ALWAYS,
changes made by both a user and an application are validated. When set
to JCTblEnum.VALIDATE_NEVER, changes are never validated.
getVisibleColumns
public int getVisibleColumns()
- Gets the table's VisibleColumns value.
- See Also:
- setVisibleColumns
setVisibleColumns
public void setVisibleColumns(int v)
- Sets the number of columns currently visible or partially visible.
This value is updated when a user interactively resizes columns or the
table. When set, the table attempts to resize to display the specified
number of columns. Setting this property to JCTblEnum.NOVALUE causes the
table to attempt to resize to make all columns visible, and sets VisibleColumns
equal to NumColumns (this happens also when creating the component if this
value is defaulted). If set to greater than NumColumns, a theoretical value
is calculated based on 10-character columns.
getVisibleRows
public int getVisibleRows()
- Gets the table's VisibleRows value.
- See Also:
- setVisibleRows
setVisibleRows
public void setVisibleRows(int v)
- Sets the number of rows currently visible or partially visible. This
value is updated when a user interactively resizes rows or the table. When
set, the table attempts to resize to display the specified number of rows.
Setting this property to JCTblEnum.NOVALUE causes the table to attempt
to resize to make all rows visible, and sets VisibleRows equal to NumRows
(this happens also when creating the component if this value is defaulted).
If set to greater than NumRows, a theoretical value is calculated based
on 1-line rows.
addColumn
public boolean addColumn(int position,
Object label,
Vector values)
- Inserts a new column into the table, shifting any cell values and attributes
(such as background color) to the right of the insertion. The column labels
will be shifted unless a LABEL_VALUE callback has been registered.
- Parameters:
- position - this is the column index before which to add the new column
(if set to JCTblEnum.MAXINT, the column is added after the last column)
- label - this is the column label (can be null)
- values - an array of objects to be inserted (can be null)
- Returns:
- false if any argument is invalid
- See Also:
- LABEL_VALUE
addRow
public boolean addRow(int position,
Object label,
Vector values)
- Inserts a new row into the table, shifting any cell values and attributes
(such as background color) below the insertion. The row labels will be
shifted unless a LABEL_VALUE callback has been registered.
- Parameters:
- position - this is the row index before which to add the new row (if
set to JCTblEnum.MAXINT, the row is added after the last row)
- label - this is the row label (can be null)
- values - an array of objects to be inserted (can be null)
- Returns:
- false if any argument is invalid
- See Also:
- LABEL_VALUE
cancelEdit
public boolean cancelEdit(boolean hide)
- Cancels interactive editing of a cell and, as an option, hides the
Text component. Any text entered or changed in the current cell by a user
is discarded.
- Parameters:
- hide - determines whether or not to hide the Text component
- Returns:
- false if no edit is in progress
commitEdit
public boolean commitEdit(boolean hide)
- Commits interactive cell editing internally, saving any changes made
by the user. The VALIDATE_CELL callback is called first to verify that
the changes are valid.
- Parameters:
- hide - determines whether to hide the Text component
- Returns:
- false if no edit is in progress
- See Also:
- VALIDATE_CELL
deleteColumns
public boolean deleteColumns(int pos,
int num_columns)
- Deletes columns from the table. The attributes (such as background
color) of the remaining columns are unaffected by the deletion. The column
labels will be shifted unless a LABEL_VALUE callback has been registered.
- Parameters:
- pos - this is the first column number to delete
- num_columns - this is the number of columns to be deleted
- Returns:
- false if any argument is invalid
- See Also:
- LABEL_VALUE
deleteRows
public boolean deleteRows(int pos,
int num_rows)
- Deletes rows from the table. The attributes (such as background color)
of the remaining rows are unaffected by the deletion. The row labels will
be shifted unless a LABEL_VALUE callback has been registered.
- Parameters:
- pos - this is the first row number to delete
- num_rows - this is the number of rows to be deleted
- Returns:
- false if any argument is invalid
- See Also:
- LABEL_VALUE
traverse
public boolean traverse(int row,
int column,
boolean show_text,
boolean select)
- Attempts to move the current cell focus to a new location. commitEdit()
is first called to commit the edit in the current cell. If commitEdit()
returns false, traverse() returns false and does not move the focus. Otherwise,
traverse() attempts to traverse to the specified cell. If the cell is not
traversable, the method returns false. The ENTER_CELL callback is called
for the cell to notify the application. If traversing to a new cell, a
2-pixel black border is drawn inside the cell to indicate that it has focus.
If traversing to the cell which currently has focus, a Text component is
displayed on top of the cell, and an ENTER_CELL callback is called with
the "stage" member set to END.
- Parameters:
- row,column - this is the index of the cell to be edited
- show_text - if this is true, the Text component is displayed in the
cell. getTextComponent can then be used to retrieve it. If false, then
the cell is highlighted.
- select - this determines whether the cell should be selected if SelectionPolicy
is not NONE (i.e., whether the SELECT callback should be called)
- Returns:
- false if any argument is invalid
- See Also:
- ENTER_CELL,
getTextComponent
getTextComponent
public TextComponent getTextComponent()
- Returns the currently displayed Text component. If no cell is being
edited, null is returned.
- See Also:
- traverse
eventToCell
public JCCellPosition eventToCell(Event event)
- Determines the cell or label in which an event occurred.
- Parameters:
- event - the event to be processed
- Returns:
- null if the event was not within a cell or label
getCurrentCell
public JCCellPosition getCurrentCell()
- Determines the cell being edited.
- Returns:
- null if there is no current cell
- See Also:
- traverse
findSpan
public int findSpan(int row,
int column,
JCCellRange range,
Dimension size)
- Finds the first range which the specified cell or label spans. Otherwise,
the first range which includes the specified cell is returned.
- Parameters:
- range - if not null, it contains the spanning cell
- size - if not null, it contains the size of the spanned range
- Returns:
- the index within the span list, or JCTblEnum.NOTFOUND if no range was
found
- See Also:
- setSpans
moveColumns
public boolean moveColumns(int source,
int num_columns,
int destination)
- Moves a range of columns. All attributes (such as the background color)
move along with the columns. The column labels will be moved unless a LABEL_VALUE
callback has been registered.
- Parameters:
- source - this is the first column in the range
- num_columns - this is the number of columns to move
- destination - this is the column number before which to insert the
moved columns
- Returns:
- false if any argument is invalid
- See Also:
- LABEL_VALUE
moveRows
public boolean moveRows(int source,
int num_rows,
int destination)
- Moves a range of rows. All attributes (such as the background color)
move along with the rows. The row labels will be shifted unless a LABEL_VALUE
callback has been registered.
- Parameters:
- source - this is the first row in the range
- num_rows - this is the number of rows to move
- destination - this is the row number before which to insert the moved
rows
- shift_labels - this determines whether to move the row labels as well
- Returns:
- false if any argument is invalid
- See Also:
- LABEL_VALUE
makeVisible
public boolean makeVisible(int row,
int col)
- This scrolls a frozen or non-frozen cell or label so it is visible
on the screen.
- Returns:
- false if an argument is invalid
makeColumnVisible
public boolean makeColumnVisible(int column)
- This scrolls a column so it is visible on the screen.
- Returns:
- false if the column is invalid
makeRowVisible
public boolean makeRowVisible(int row)
- This scrolls a row so it is visible on the screen.
- Returns:
- false if the row is invalid
isVisible
public boolean isVisible(int row,
int col)
- This checks whether a cell is visible.
getColumnLabel
public Object getColumnLabel(int column)
- This gets a column label. To get the AWT component currently in the
label, call getComponent.
- See Also:
- getComponent
getRowLabel
public Object getRowLabel(int row)
- This gets a row label. To get the AWT component currently in the label,
call getComponent.
- See Also:
- getComponent
getCell
public Object getCell(int row,
int col)
- This gets the value of a cell or label. To get the AWT component currently
in the cell, call getComponent.
- See Also:
getComponent
sortByColumn
public boolean sortByColumn(int column,
JCSortInterface sort_if)
- Sorts the rows in the table based on a specified column, using the
String equivalents of cell values. It does not sort cell values supplied
by the CELL_VALUE callback. Calling this method has no effect on the spanned
ranges, cell properties or selected cells of the table, as only the values
stored in the table are changed. After the table has been sorted, the SORT
callback is called.
- Parameters:
- sort_if - An optional interface whose compare method is called during
sorting, to provide a mechanism for the application to specify whether
one object is greater than another (similar to qsort's compare function).
If this parameter is null, the column is sorted by numerical or string
comparison as applicable.
- See Also:
- JCSortInterface,
CELL_VALUE, SORT
makeVisible
public boolean makeVisible(int row,
int col)
- This scrolls a frozen or non-frozen cell or label so it is visible
on the screen.
- Returns:
false if an argument is invalid
mouseDown
public boolean mouseDown(Event event,
int x,
int y)
- If the SHIFT key or CONTROL key is pressed, a new range is selected.
If currently over an URL anchor, returns.
Otherwise, resizes a cell, traverses, sorts or selects.
- See Also:
- RESIZE_CELL,
SELECT, SORT,
TRAVERSE_CELL
mouseDrag
public boolean mouseDrag(Event event,
int x,
int y)
- Resizes a cell or selects it.
- See Also:
- RESIZE_CELL,
SELECT
mouseExit
public boolean mouseExit(Event event,
int x,
int y)
- This tracks the cursor if TrackCursor is true.
- Overrides:
- mouseExit
in class Component
- See Also:
setTrackCursor
mouseMove
public boolean mouseMove(Event event,
int x,
int y)
- This tracks the cursor if TrackCursor is true.
- Overrides:
- mouseMove
in class Component
- See Also:
setTrackCursor
mouseUp
public boolean mouseUp(Event event,
int x,
int y)
- Resizes a cell or selects it. If the cursor is currently over an URL
anchor, it displays it.
- See Also:
- RESIZE_CELL,
SELECT
preferredSize
public Dimension preferredSize()
Returns the preferred size of the component.
- Overrides:
- preferredSize
in class Container
keyDown
public boolean keyDown(Event ev,
int key)
- If key is PGDN or PGUP, the table is scrolled.
If key is LEFT, RIGHT, DOWN, UP, TAB, or SHIFT-TAB, the adjacent cell
is traversed to. If key is ESC, current resizing, selecting or editing
is cancelled.
- See Also:
- RESIZE_CELL,
SCROLL, SELECT,
SORT, TRAVERSE_CELL,
setMode
getBounds
public boolean getBounds(int row,
int col,
Rectangle bounds)
- Gets the bounding rectangle of a cell or label.
- Parameters:
- row,col - cell location
- bounds - returned position and size
- Returns:
- false if cell or label does not exist
isSelected
public boolean isSelected(int row,
int col)
- Determines whether the cell is selected.
isTraversable
public boolean isTraversable(int row,
int col)
- Returns true if the cell can be traversed to -- in other words, if
its traverse property is true, and is not spanned by another cell.
- See Also:
- setTraversable, setSpans
getColumnLabels
public Vector getColumnLabels()
- Gets the table's ColumnLabels value.
- See Also:
- setColumnLabels
getRowLabels
public Vector getRowLabels()
- Gets the table's RowLabels value.
- See Also:
- setRowLabels
getColumnLabelPlacement
public int getColumnLabelPlacement()
- Gets the table's ColumnLabelPlacement value.
- See Also:
- setColumnLabelPlacement
setColumnLabelPlacement
public void setColumnLabelPlacement(int v)
- Sets the position of the column labels relative to the cells. Valid
values are JCTblEnum.PLACE_TOP (default) and JCTblEnum.PLACE_BOTTOM.
getColumnLabelSort
public boolean getColumnLabelSort()
- Gets the table's ColumnLabelSort value.
- See Also:
- setColumnLabelSort
setColumnLabelSort
public void setColumnLabelSort(boolean v)
- Specifies whether or not a column is sorted when its label is clicked
(default: false). If true, sortByColumn() will be called, and the column
will not be selected regardless of the value of SelectionPolicy.
- See Also:
- setSelectionPolicy, sortByColumn
getRowLabelOffset
public int getRowLabelOffset()
- Gets the table's RowLabelOffset value.
- See Also:
- setRowLabelOffset
setRowLabelOffset
public void setRowLabelOffset(int v)
- Sets the spacing between the row labels and the cell area in pixels
(default: 0). If a negative value is specified, the labels will overlap
the cell area.
getColumnLabelOffset
public int getColumnLabelOffset()
- Gets the table's ColumnLabelOffset value.
- See Also:
- setColumnLabelOffset
setColumnLabelOffset
public void setColumnLabelOffset(int v)
- Sets the spacing between the column labels and the cell area in pixels
(default: 0). If a negative value is specified, the labels will overlap
the cell area.
getRowLabelPlacement
public int getRowLabelPlacement()
- Gets the table's RowLabelPlacement value.
- See Also:
- setRowLabelPlacement
setRowLabelPlacement
public void setRowLabelPlacement(int v)
- Sets the position of the row labels relative to the cells. Valid values
are JCTblEnum.PLACE_LEFT (default) and PLACE_RIGHT.
clearCells
public void clearCells()
- Sets clears the values of all cells by setting them to null.
- See Also:
- setCells
setCells
public void setCells(String v[][])
- Sets the cell values as a matrix of Strings.
To remove all values, call clearCells().
- See Also:
- clearCells
setCells
public void setCells(Vector v)
- Sets the cell values as a Vector of Vectors. Each element of the vector
may be an instance of a String, JCString, Image, or other Object. If an
Object, the instance's toString() method is used to get a text representation
for display.
To remove all values, call clearCells.
To set an AWT component in a cell, call setComponent
- See Also:
- setComponent, clearCells
getCells
public JCVector getCells()
- Returns the internal cell values.
- See Also:
- setCells
setColumnLabels
public void setColumnLabels(Vector labels)
- Sets the column labels as a Vector. Each element of the vector may
be an instance of a String, JCString, Image, Component or other Object.
If an Object, the instance's toString() method will be used to get a text
representation for display. To clear the labels, call this method with
a null argument.
setColumnLabels
public void setColumnLabels(String labels[])
- Sets the column labels as an array of Strings.
setColumnLabel
public void setColumnLabel(int column,
Object v)
- Sets a column label. The element may be an instance of a String, JCString, Image, Component or other Object. If an Object, the instance's toString() method will be used to get a text representation for display. To clear the label, call this method with a null argument.
setRowLabels
public void setRowLabels(Vector labels)
- Sets the row labels as a Vector. Each element of the vector may be
an instance of a String, JCString, Image, Component or other Object. If
an Object, the instance's toString() method will be used to get a text
representation for display. To clear the labels, call this method with
a null argument.
setRowLabels
public void setRowLabels(String labels[])
- Sets the row labels as an array of Strings.
setRowLabel
public void setRowLabel(int row,
Object v)
- Sets a row label. The element may be an instance of a String, JCString, Image, Component or other Object. If an Object, the instance's toString() method will be used to get a text representation for display. To clear the label, call this method with a null argument.
setCell
public void setCell(int row,
int col,
Object v)
- Sets the value of a cell or label.
setAlignment
public void setAlignment(int row,
int col,
int v)
- Sets the alignment of cell/label text and/or image. Valid values are:
JCTblEnum.TOPLEFT, JCTblEnum.TOPCENTER, JCTblEnum.TOPRIGHT, JCTblEnum.MIDDLELEFT,
JCTblEnum.MIDDLECENTER, JCTblEnum.MIDDLERIGHT, JCTblEnum.BOTTOMLEFT, JCTblEnum.BOTTOMCENTER
and JCTblEnum.BOTTOMRIGHT
setBackground
public void setBackground(int row,
int col,
Color v)
- Sets the background color of cells and labels. When set to null the
color is inherited from Component's background value.
setBackground
public void setBackground(int row,
int col,
int v)
- Allows the background color of a cell or label to be set to JCTblEnum.REPEAT_ROW
or JCTblEnum.REPEAT_COLUMN. The color is then taken from the list specified
by RepeatBackgroundColors, repeating in row or column order.
- See Also:
- setRepeatBackgroundColors
setBorderSides
public void setBorderSides(int row,
int col,
int v)
- Sets the sides of a cell or label that display the border type in their
border area. The value is a bitwise OR of the following: JCTblEnum.BORDERSIDE_NONE,
JCTblEnum.BORDERSIDE_ALL, JCTblEnum.BORDERSIDE_LEFT, JCTblEnum.BORDERSIDE_RIGHT,
JCTblEnum.BORDERSIDE_TOP, JCTblEnum.BORDERSIDE_BOTTOM The width of all
cell/label borders is specified by ShadowThickness.
setBorderType
public void setBorderType(int row,
int col,
int v)
- Sets the style used for cell and label borders. Valid values are:
JCTblEnum.BORDER_ETCHED_IN double line; cell appears inset
JCTblEnum.BORDER_ETCHED_OUT double line; cell appears raised
JCTblEnum.BORDER_FRAME_IN 1-pixel shadow-in at edge; cell appears framed
JCTblEnum.BORDER_FRAME_OUT 1-pixel shadow-out at edge; cell appears framed
JCTblEnum.BORDER_IN cell appears inset
JCTblEnum.BORDER_OUT cell appears raised
JCTblEnum.BORDER_PLAIN shadow drawn in foreground color
JCTblEnum.BORDER_NONE no border
The width of all cell/label borders is specified by ShadowThickness.
ShadowThickness must be greater than 5 pixels to see the effects of the
ETCHED and FRAME borders.
- See Also:
- setShadowThickness
setCharHeight
public void setCharHeight(int row,
int v)
- Sets the number of lines of text that a row can display (default: 1).
Multi-line cell values that do not fit in a cell or label are clipped.
This property controls the height only if pixelHeight is JCTblEnum.NOVALUE.
This property works best with lists that use fixed-width fonts. When a
user interactively resizes a row, this property is overridden by the pixel
height. pixelHeight updates to specify the pixel height of the user-resized
row.
- Parameters:
- row - row whose height is to be set. This may be a specific row, JCTblEnum.LABEL
(set height of column labels), or ALL (set height of all rows).
- See Also:
- setPixelHeight
setCharWidth
public void setCharWidth(int col,
int v)
- Sets the number of characters that a column can display (default: 10).
Cell values that do not fit in a cell or label are clipped. This property
controls the width only if PixelWidth is JCTblEnum.NOVALUE. This property
works best with lists that use fixed-width fonts. When a user interactively
resizes a column, this property is overridden by the pixelWidth. pixelWidth
updates to specify the pixel width of the user-resized column.
- Parameters:
- col - column whose width is to be set. This may be a specific column,
JCTblEnum.LABEL (set width of row labels), or ALL (set width of all columns).
- See Also:
- setPixelWidth
getComponent
public Component getComponent(int row,
int col)
- Locates the component currently displayed in the cell, and returns
null if there is no component in the cell or if the component is not currently
displayed.
- Parameters:
- row,col - the cell or label position to be found
- See Also:
- setComponent
getPosition
public JCCellPosition getPosition(Component component,
JCCellPosition pos)
- Finds the location of any component set in a cell/label.
- Parameters:
- component - the component whose location is to be found, which may
be a child of a component in a cell.
- pos - the object to be used to return the position, or null
- Returns:
- if pos is null, the position is returned; otherwise null. If the component
is not currently displayed, pos.row will be set to JCTblEnum.NOVALUE (if
pos is not null) and null is returned.
- See Also:
- setComponent
setComponent
public void setComponent(int row,
int col,
Component comp)
- Sets the component for a cell or label.
setDatatype
public void setDatatype(int row,
int col,
int v)
- Sets the data type of a cell/label value. When set, this property affects
cell and/or label value changes from that point on. Before committing a
changed cell/label value, the table additionally calls the VALIDATE_CELL
callback if one has been registered.
Valid data types: JCTblEnum.TYPE_STRING, TYPE_DOUBLE, TYPE_INTEGER,
TYPE_FLOAT, and TYPE_BOOLEAN.
getEditable
public boolean getEditable(int row,
int col)
- Returns the editable value for a cell.
- See Also:
- setEditable
setEditable
public void setEditable(int row,
int col,
boolean v)
- Sets whether cell values can be changed by an end-user (default: true).
setFont
public void setFont(int row,
int col,
Font v)
- Sets the font to be used to display cell and/or label values.
setForeground
public void setForeground(int row,
int col,
Color v)
- Sets the foreground color (the text color) of cells and labels. When
set to null the table's foreground value is used.
setForeground
public void setForeground(int row,
int col,
int v)
- Allows the foreground color of a cell or label to be set to JCTblEnum.REPEAT_ROW
or JCTblEnum.REPEAT_COLUMN. The color is then taken from the list specified
by RepeatForegroundColors, repeating in row or column order.
- See Also:
- setRepeatForegroundColors
getMaxLength
public int getMaxLength(int row,
int col)
- Returns the maxLength value for a cell.
- See Also:
setMaxLength
public void setMaxLength(int row,
int col,
int v)
- Sets the maximum number of characters that can be entered in a cell
by a user (default: MAXINT). This property does not affect cell values
changed with setCells() and setCell(). Newline characters in multi-line
cells count as one character.
- See Also:
- setCell, setCells
getMultiline
public boolean getMultiline(int row,
int col)
- Returns the multiline value for a cell.
- See Also:
- setMultiline
setMultiline
public void setMultiline(int row,
int col,
boolean v)
- Sets whether or not a user can enter multiple lines of text into a
cell (default: false). This property does not affect cell values added
or changed by the application. If the cell's value is already multiple
lines, a TextArea component is displayed when the cell is edited, regardless
of the multiline value.
setPixelHeight
public void setPixelHeight(int row,
int v)
- Sets a row height in pixels. Cell values, images, or components that
do not fit vertically in a cell are clipped. This property controls the
height unless it is set to JCTblEnum.NOVALUE -- charHeight then controls
the height.
The following special values are valid:
JCTblEnum.VARIABLE set to the highest value in the row
JCTblEnum.AS_IS do not change
When a user interactively resizes a row, this property updates. If character
specification was in effect for the row, it is overridden by the pixel
height of the user-resized row.
- Parameters:
- row - row whose height is to be set. This may be a specific row, JCTblEnum.LABEL
(set height of column labels), or ALL (set height of all rows).
- See Also:
- setCharHeight
setPixelWidth
public void setPixelWidth(int col,
int v)
- Sets a column width in pixels. Cell values, images, or components that
do not fit vertically in a cell are clipped. This property controls the
width unless it is set to JCTblEnum.NOVALUE - charWidth then controls the
width.
The following special values are valid:
JCTblEnum.VARIABLE set to the highest value in the column
JCTblEnum.AS_IS do not change
When a user interactively resizes a column, this property updates. If
character specification was in effect for the column, it is overridden
by the pixel width of the user-resized column.
- Parameters:
- col - column whose width is to be set. This may be a specific column,
JCTblEnum.LABEL (set width of row labels), or ALL (set width of all columns).
- See Also:
- setCharWidth
getStringCase
public int getStringCase(int row,
int col)
- Returns the stringCase value for a cell.
- See Also:
- setStringCase
setStringCase
public void setStringCase(int row,
int col,
int v)
- Sets the case of cell values of cells edited by the user. This property
does not convert the case of existing cell values. This property is only
used when the cell's DataType is JCTblEnum.TYPE_STRING. Valid values are:
JCTblEnum.CASE_LOWER Convert to lower case
JCTblEnum.CASE_AS_IS No conversion
JCTblEnum.CASE_UPPER Convert to upper case
getTraversable
public boolean getTraversable(int row,
int col)
- Returns the traversable value for a cell.
- See Also:
- setTraversable
setTraversable
public void setTraversable(int row,
int col,
boolean v)
- Sets whether the cell can be traversed to (default: true).
getUserdata
public Object getUserdata(int row,
int col)
- Returns the userdata object for a cell or label.
- See Also:
- setUserdata
setUserdata
public void setUserdata(int row,
int col,
Object v)
- Sets a user data object that the application can attach. This property
is not used by JCTable.
All Packages Class Hierarchy This Package Previous Next Index